🐍 How to fix [Errno 2] No such file or directory: 'requirements.txt' in Python
![🐍 How to fix [Errno 2] No such file or directory: 'requirements.txt' in Python](/content/images/size/w960/2024/08/R.jpeg)
The Problem: A Mysterious FileNotFoundError
When building a Python package, you might encounter an unexpected error:
FileNotFoundError: [Errno 2] No such file or directory: 'requirements.txt'
This error can be particularly frustrating when you're certain that the requirements.txt
file exists in your project directory.
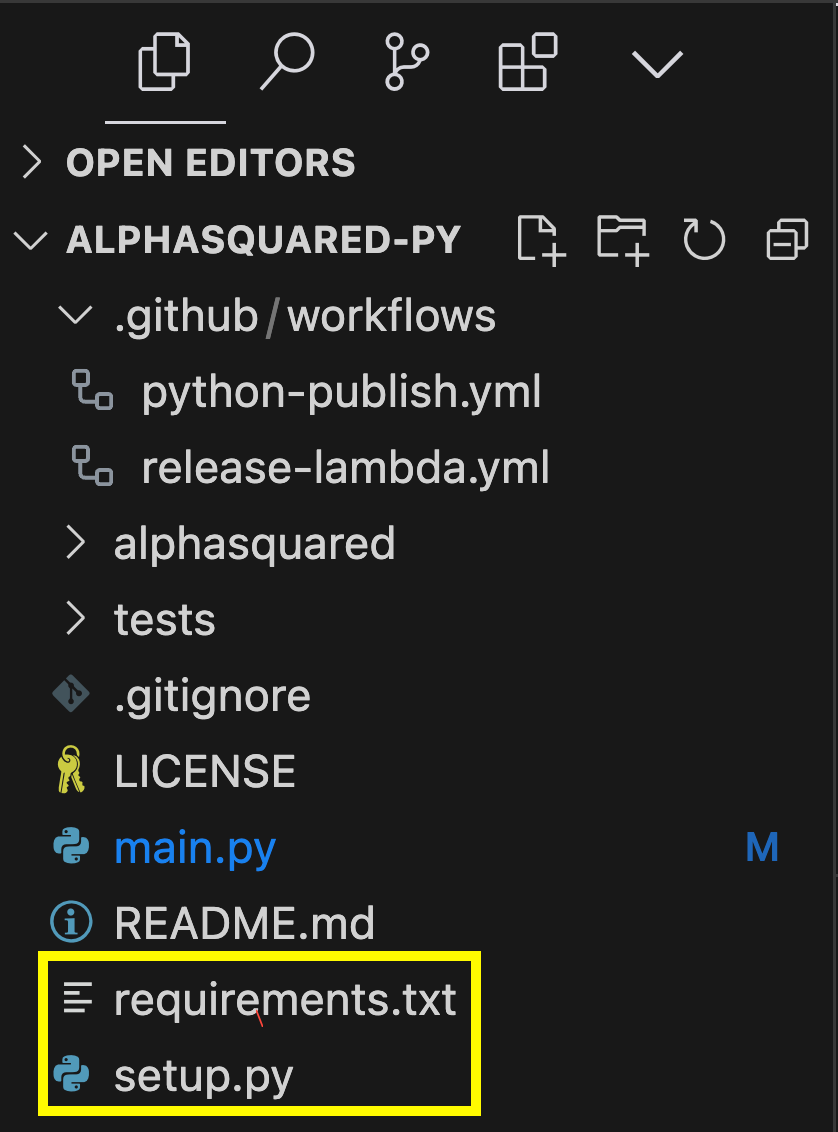
Upon closer inspection, we noticed that the error occurred during the wheel-building process, which takes place in a temporary directory. This directory is created by the build system and doesn't include all the files from your project root.
* Getting build dependencies for wheel...
Python version: 3.11.7 (main, Dec 4 2023, 18:10:11) [Clang 15.0.0 (clang-1500.1.0.2.5)]
Current working directory: /private/var/folders/nv/gl8r1jk11lgdplczpvczqjfc0000gn/T/build-via-sdist-pfcgxrbo/alphasquared_py-0.1.0
Contents of current directory: ['PKG-INFO', 'alphasquared_py.egg-info', 'LICENSE', 'tests', 'README.md', 'alphasquared', 'setup.py', 'setup.cfg']
Looking for requirements.txt at: /private/var/folders/nv/gl8r1jk11lgdplczpvczqjfc0000gn/T/build-via-sdist-pfcgxrbo/alphasquared_py-0.1.0/requirements.txt
requirements.txt not found at /private/var/folders/nv/gl8r1jk11lgdplczpvczqjfc0000gn/T/build-via-sdist-pfcgxrbo/alphasquared_py-0.1.0/requirements.txt
The issue stems from how Python's setuptools handles file inclusion during the build process. By default, setuptools only includes Python modules and packages. Other files, like requirements.txt
, aren't automatically included in the distribution.
The Solution: MANIFEST.in to the Rescue
The solution to this problem is to use a MANIFEST.in
file. This file tells setuptools which additional files to include in the source distribution.
Here's what our MANIFEST.in
file looks like:
include requirements.txt
include README.md
include LICENSE
recursive-include alphasquared *.py
recursive-include tests *.py
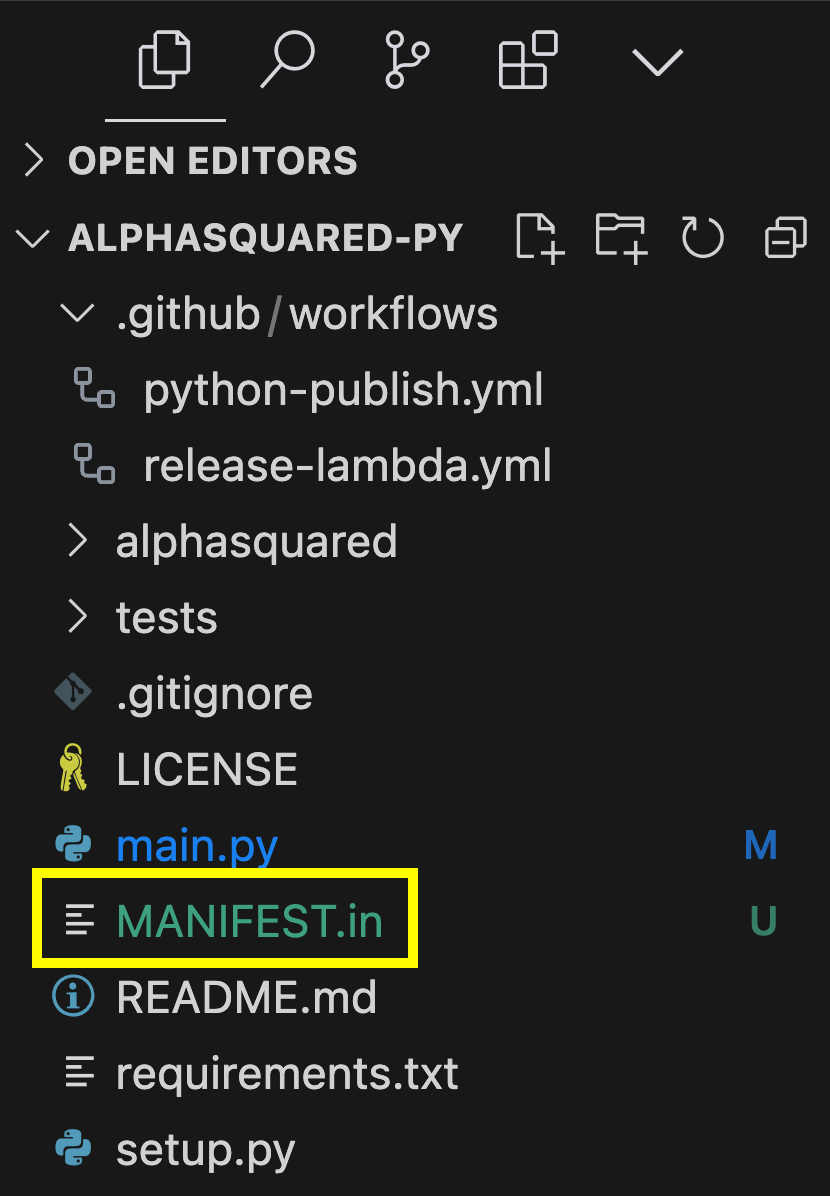
Why This Works
The MANIFEST.in
file works because it explicitly tells setuptools to include requirements.txt
(along with other important files) in the source distribution. This ensures that when the wheel is being built in the temporary directory, requirements.txt
is available.
However, just creating the MANIFEST.in
file isn't enough. We also need to tell setuptools to use it. This is done in the setup.py
file:
setup(
# ... other parameters ...
include_package_data=True,
# ... other parameters ...
)
The include_package_data=True
parameter tells setuptools to read the MANIFEST.in
file and include the specified files in the package distribution.
The Result
After adding the MANIFEST.in
file and ensuring include_package_data=True
in setup.py
, the build process succeeds without the FileNotFoundError.
* Getting build dependencies for wheel...
/private/var/folders/nv/gl8r1jk11lgdplczpvczqjfc0000gn/T/build-env-1qaxzj4r/lib/python3.11/site-packages/setuptools/_distutils/dist.py:268: UserWarning: Unknown distribution option: 'test_suite'
warnings.warn(msg)
running egg_info
writing alphasquared_py.egg-info/PKG-INFO
writing dependency_links to alphasquared_py.egg-info/dependency_links.txt
writing requirements to alphasquared_py.egg-info/requires.txt
writing top-level names to alphasquared_py.egg-info/top_level.txt
reading manifest file 'alphasquared_py.egg-info/SOURCES.txt'
reading manifest template 'MANIFEST.in'
adding license file 'LICENSE'
writing manifest file 'alphasquared_py.egg-info/SOURCES.txt'
* Building wheel...
/private/var/folders/nv/gl8r1jk11lgdplczpvczqjfc0000gn/T/build-env-1qaxzj4r/lib/python3.11/site-packages/setuptools/_distutils/dist.py:268: UserWarning: Unknown distribution option: 'test_suite'
warnings.warn(msg)
running bdist_wheel
running build
running build_py
creating build
creating build/lib
creating build/lib/alphasquared
copying alphasquared/__init__.py -> build/lib/alphasquared
copying alphasquared/alphasquared.py -> build/lib/alphasquared
running egg_info
writing alphasquared_py.egg-info/PKG-INFO
writing dependency_links to alphasquared_py.egg-info/dependency_links.txt
writing requirements to alphasquared_py.egg-info/requires.txt
writing top-level names to alphasquared_py.egg-info/top_level.txt
reading manifest file 'alphasquared_py.egg-info/SOURCES.txt'
reading manifest template 'MANIFEST.in'
adding license file 'LICENSE'
writing manifest file 'alphasquared_py.egg-info/SOURCES.txt'
installing to build/bdist.macosx-14-arm64/wheel
running install
running install_lib
creating build/bdist.macosx-14-arm64
creating build/bdist.macosx-14-arm64/wheel
creating build/bdist.macosx-14-arm64/wheel/alphasquared
copying build/lib/alphasquared/__init__.py -> build/bdist.macosx-14-arm64/wheel/./alphasquared
copying build/lib/alphasquared/alphasquared.py -> build/bdist.macosx-14-arm64/wheel/./alphasquared
running install_egg_info
Copying alphasquared_py.egg-info to build/bdist.macosx-14-arm64/wheel/./alphasquared_py-0.1.0-py3.11.egg-info
running install_scripts
creating build/bdist.macosx-14-arm64/wheel/alphasquared_py-0.1.0.dist-info/WHEEL
creating '/Users/rhettreisman/Documents/GitHub/alphasquared-py/dist/.tmp-skyupiwf/alphasquared_py-0.1.0-py3-none-any.whl' and
adding 'build/bdist.macosx-14-arm64/wheel' to it
adding 'alphasquared/__init__.py'
adding 'alphasquared/alphasquared.py'
adding 'alphasquared_py-0.1.0.dist-info/LICENSE'
adding 'alphasquared_py-0.1.0.dist-info/METADATA'
adding 'alphasquared_py-0.1.0.dist-info/WHEEL'
adding 'alphasquared_py-0.1.0.dist-info/top_level.txt'
adding 'alphasquared_py-0.1.0.dist-info/RECORD'
removing build/bdist.macosx-14-arm64/wheel
Successfully built alphasquared_py-0.1.0.tar.gz and alphasquared_py-0.1.0-py3-none-any.whl
Successful build after including MANIFEST
Lessons Learned
- Understand the build process: The Python package build process involves creating temporary directories. Files that aren't Python modules might not be automatically included.
- Use MANIFEST.in: For non-Python files that need to be included in your distribution, use a
MANIFEST.in
file to explicitly specify them. - Configure setup.py correctly: Make sure to set
include_package_data=True
in yoursetup()
function to tell setuptools to use yourMANIFEST.in
file. - Debug with verbose output: When troubleshooting build issues, use the
--verbose
flag with your build command to get more detailed information about what's happening during the build process.
By understanding these aspects of the Python packaging process, you can avoid similar issues in your own projects and create more robust, distributable packages.
I diagnosed and fixed this issue using Cursor.sh (the easiest to use AI developer assistant). You can learn more about it here:
Cursor Tutorial